I’ve mentioned previously about How to Create a Random Password using the .NET Framework.
That works for Windows Powershell, but it doesn’t work for .NET 5, which is what modern versions of PowerShell use.
So what can you do?
Well, thankfully it’s pretty easy to write a simple random password generator in PowerShell 7. It follows a simple pattern, and I’ll show you how to do it.
- Make a list of characters
- Decide how long the password is going to be
- Use a For-Loop to add characters to an array
Make your list of characters
You have a few options here. You could do something like this:
# Take a string of acceptable characters and change it into an array of individual characters
[char[]]"abcdefABCDEF1234567890"
You could also use the RANGE selector (..) to help you
'a'..'z'
If you want to use multiple RANGES, you can do that too. Ranges can be added together to form a bigger list:
'a'..'z' + 'A'..'Z' + '0'..'9'
I actually wanted to include some special characters in my list, but I didn’t want to have any special characters that might cause problems when used in a SQL Server password.
Showing the list of ASCII characters

There are 255 ASCII characters. Some of them (like ♣ or ?) would not be useful to me for a password.
But a quick list like this would help me to find the ranges that I want to include for my password generator.
0..255 | Foreach-Object {"$_ : $([char]$_)"}
#In English, this is:
# Give me a list of numbers from 0 to 255.
# Then for each of those numbers, write this to the screen:
# The Number, a colon, and the ASCII character equivalent for that number
After seeing the characters in a list, and checking to make sure I wasn’t going to use any special characters that would cause me problems later, this is what I ended up with.
$charlist = [char]94..[char]126 + [char]65..[char]90 + [char]47..[char]57
# All uppercase and lowercase letters, all numbers and some special characters.
No matter which way you create your list, you should end up with a variable to hold your characters. It should be a variable that is a character array.
$charList.GetType()
Should confirm that you have an array, not a string.
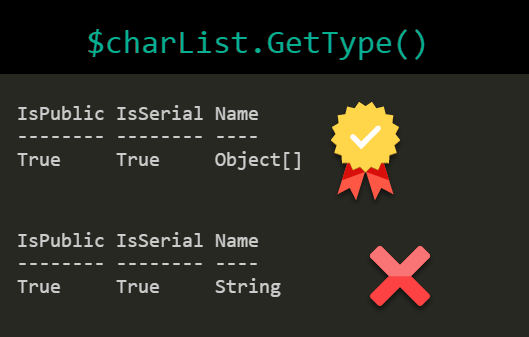
Got it so far? The rest is easy!
Decide how long you want your password to be
I’m not going to ever try to remember this password.
It’s mostly going to be:
- Generated
- Saved in an Azure KeyVault
- Looked up later when I need it
So I don’t really care what it is as long as it’s complex, long and impossible to guess.
But I don’t want it to even have the same number of characters each time.
I’ll have a range of password lengths. I want passwords to be at least 20 characters long, but it doesn’t need to be longer than 32.
There are a few logical ways to do that.
- A random number between 0 and 12, plus 20
- A random number between 20 and 32
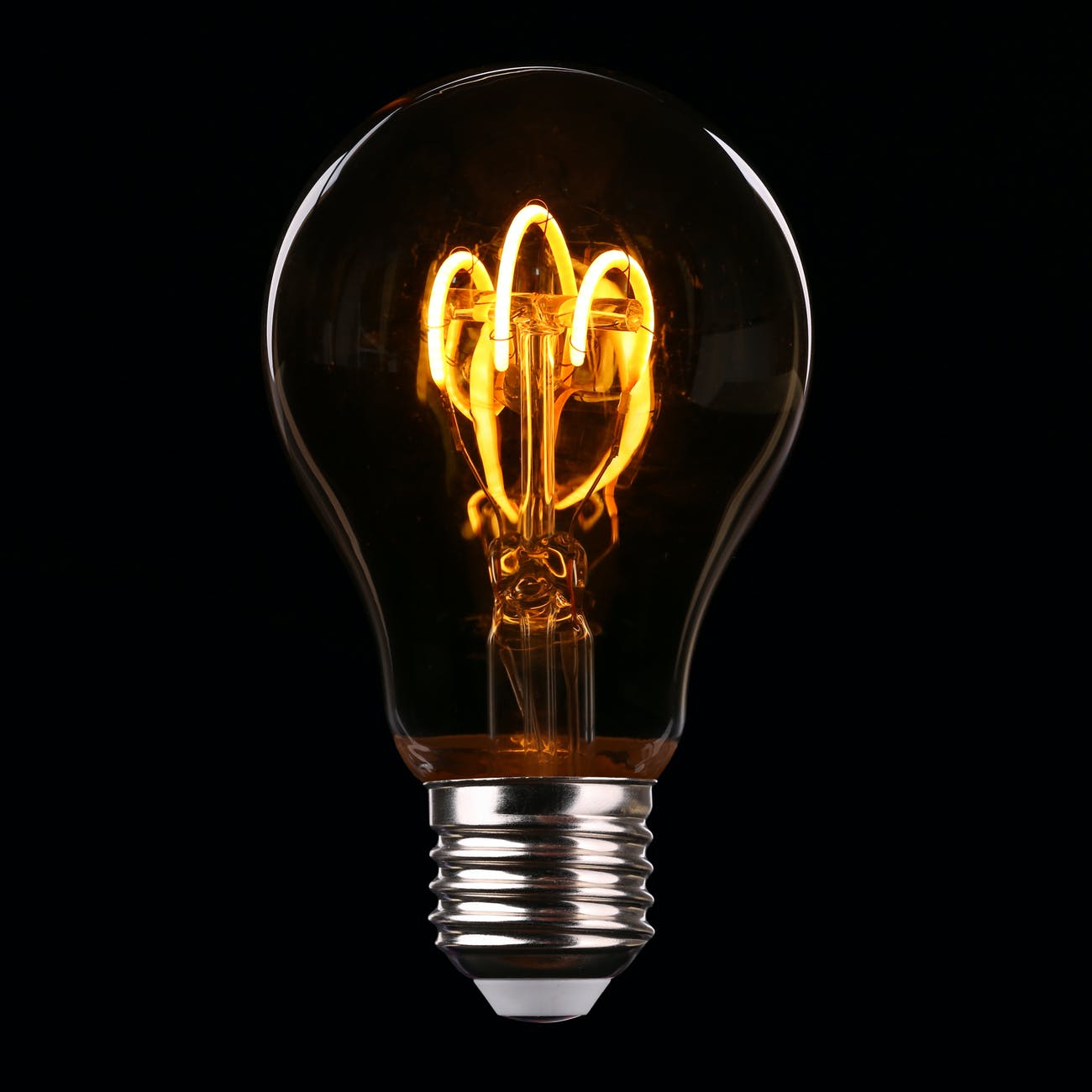
When I first wrote this, my brain was still thinking about “ranges” from character selection.
But there was a better way…
All of these lines do the same thing: They give me a random number between 20 and 32.
# Option 1: Randomize the variation
$PwdLength = ([0..12] | Get-Random) + 20
# Option 2: Randomization from the whole range
$PwdLength = [20..32] | Get-Random
#Option 3: Built in parameters from a native PowerShell cmdlet.
$PwdLength = Get-Random -Minimum 20 -Maximum 32
Option 3 is best. It’s the easiest one to read.
Now make your random password from the list of characters
#Create a new empty array to store the list of random characters
$pwdList = @()
# Use a FOR loop to pick a character from the list one time for each count of the password length
For ($i = 0; $i -lt $pwlength; $i++) {
$pwdList += $charList | Get-Random
}
# Join all the individual characters together into one string using the -JOIN operator
$pass = -join $pwdList
The whole thing put all together
$charlist = [char]94..[char]126 + [char]65..[char]90 + [char]47..[char]57
$pwLength = (1..10 | Get-Random) + 24
$pwdList = @()
For ($i = 0; $i -lt $pwlength; $i++) {
$pwdList += $charList | Get-Random
}
$pass = -join $pwdList